In order to examine the basic cryptographic mechanisms, I created the short python codes to generate the old encryption keys for basic encryption (decryption) algorithms. They are split to symmetric and asymmetric based on their context. Based on their structure they can be categorized to the block or stream kind. Other metrics differentiate algorithms: encryption time, decryption time, avalanche effect (algorithm strength), entropy (randomness), number of bits used, computational cost (CPU and RAM) of generating a new key pair, computational cost of generating a signature, computational cost of verifying a signature, size of private key, size of public key, signature size, etc. There are 3 main steps in the text encryption: 1. create a text, 2. create a key, 3. encrypt the message or password.
The full python code is available here. It works very good for Google Colaboratory.
1. AES (Rijndael)
Cipher type is symmetric and security is excellent. AES is very fast. Block size is 128 bits. Average number of bits needed is 256. Execution time: 0:00:00.001501 seconds.
2. RSA (Ron Rivest, Adi Shamir and Leonard Adleman)
RSA is a very popular algorithm, asymmetric, has 1 round, block size is variable, speed is the slowest among the encryption algorithms. It is the least secure and memory most expensive algorithm if implemented incorrectly. Average number of bits needed is 44. Execution time: 0:00:01.724113 seconds.
3. DSA
Asymmetric, DSA is a type of public-key encryption algorithm, it is used to generate an electronic signature. Execution time: 0:00:01.202864 seconds.
4. Salsa20
Symmetric stream cipher, well-designed, effective, approved by ESTREAM. A nonce is a random single use number to ensure that old communication is not reused. Execution time: 0:00:00.000950 seconds.
5. Chacha20
Similar to Salsa20, designed by Daniel J. Bernstein, symmetric and uses a single key to encrypt and decrypt the data. Execution time: 0:00:00.001153 seconds.
6. DES
Encrypting algorithm created by IBM in 1975. There is 16 rounds and block size is 64 bits. Cypher type is a symmetric algorithm block cipher. It is slow algorithm, not secure enough. Data encryption standard (DES) is using 56 encryption bits key length, the key length was reduced from 128 bits to 56 bits by the NSA. Average number of bits needed is 27. Execution time: 0:00:00.001569 seconds.
7. 3DES ( triple DES)
Uses 3 keys to achieve the greatest level of security. 3DES was invented by IBM in 1978, has 168 Key length. there is a 48 rounds and block size is 64 bits. It is very slow and security is sufficient. Triple DES is one of the strongest symmetric algorithms. Average number of bits needed is 40. Execution time: 0:00:00.001945 seconds.
8. RC2
RC2 (ARC2) is a symmetric key block cipher algorithm designed by Ron Rivest in 1987, in year 1996 it went public. Execution time: 0:00:00.000713 seconds.
9. Blowfish
Blowfish is a symmetric-key block cipher, designed in 1993 by Bruce Schneier and included in many cipher suites and encryption products. Blowfish provides a good encryption rate in software, and no effective cryptanalysis of it has been found to date. It is a predecessor of Twofish. Low memory needed. Average number of bits needed is 128. Execution time: 0:00:00.002014 seconds.
10. Twofish
A symmetric key block cipher with a block size of 128 bits and key sizes up to 256 bits. It was one of the five finalists of the Advanced Encryption Standard contest, but it was not selected. Twofish is related to the earlier block cipher Blowfish, in example only 16 characters secret key, execution time: 0:00:00.000434 seconds.
11. ECC (elliptic curve cryptography )
ECC is more secure than RSA, requires much shorter key lengths compared to RSA, ECC has the same security level. ECC finds a distinct logarithm within a random elliptic curve, uses x point, y point and d. ECC is the most interesting from algorithms above, usually considered as assymetric.
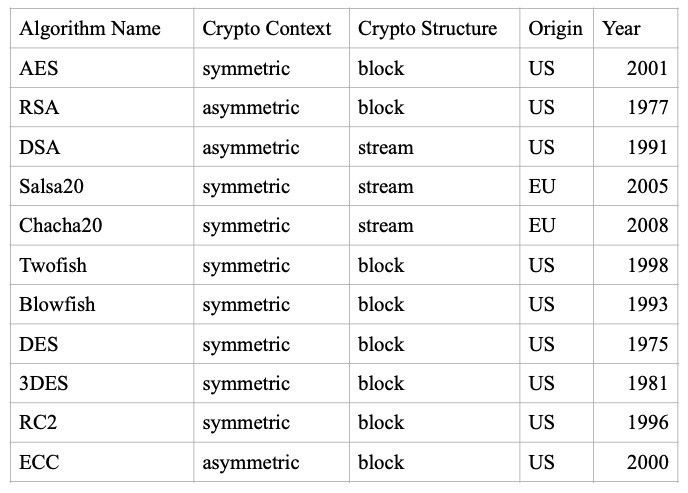
Other more recent encryption and decryption algorithms and frameworks as published by NIST Post-Quantum Cryptography Standardization are falcon, RC6, Sike, Rainbow, Classic McEliece, CRYSTALS-KYBER, FrodoKEM, SIKE, Picnic, Rabbit, Clefia, Edie, Quad, Saber, qTESLA, Bike, Bcrypt, etc. Their structure is more complex and can be used usually in the symmetric and asymmetric contexts both.
Dictionary
Python code
References
https://pycryptodome.readthedocs.io/en/latest/src/public_key/dsa.html
https://nvlpubs.nist.gov/nistpubs/ir/2022/NIST.IR.8413.pdf
https://pq-crystals.org/kyber/index.shtml
https://www.garykessler.net/library/crypto.html
https://www.nist.gov/news-events/news/2022/07/nist-announces-first-four-quantum-resistant-cryptographic-algorithms
https://iaeme.com/MasterAdmin/Journal_uploads/IJEET/VOLUME_11_ISSUE_2/IJEET_11_02_022.pdf
https://discuss.python.org/t/i-need-a-fast-elliptic-curve-cryptography-library/16787
https://medium.com/asecuritysite-when-bob-met-alice/elliptic-curve-keys-pythonand-hazmat-43750ec56ce3
https://github.com/lc6chang/ecc-pycrypto
https://stackoverflow.com/questions/50538729/how-to-encrypt-messages-with-ecc-in-pycryptodom
https://www.semanticscholar.org/paper/Enhanced-Data-Integrity-Encryption-Algorithm-for-Mothlabeng-Mathonsi/edce2e31f8dab1da2229e1105f2350c484e13e06
https://pycryptodome.readthedocs.io/en/latest/src/public_key/ecc.html
https://ww1.microchip.com/downloads/en/DeviceDoc/00003442A.pdf
https://en.wikipedia.org/wiki/Kyber
https://medium.com/asecuritysite-when-bob-met-alice/building-for-a-secure-future-goodbye-ecc-and-hello-to-saber-kyber-ntru-or-mceliece-or-even-3aa058d1d399
https://helstrom-quantum-centroid-classifier.readthedocs.io/en/latest/auto_examples/index.html
https://en.wikipedia.org/wiki/Elliptic_Curve_Digital_Signature_Algorithm
https://github.com/tprest/falcon.py/blob/master/falcon.py
https://github.com/mauricelambert/RC6Encryption/blob/main/PKG-INFO
https://www.baeldung.com/cs/stream-cipher-vs-block-cipher
https://github.com/tprest/falcon.py
https://pycryptodome.readthedocs.io/en/latest/src/cipher/chacha20.html
https://pqc-hqc.org
https://en.wikipedia.org/wiki/Ring_learning_with_errors
https://sing.stanford.edu/site/publications/kiningham19falcon.pdf
https://www.w3schools.in/cyber-security/modern-encryption
https://pypi.org/project/twofish/
https://symbiosisonlinepublishing.com/computer-science-technology/computerscience-information-technology32.php
https://www.pqcrainbow.org
https://www.cs.bham.ac.uk/~axb1471/QCSseminar.pdf
https://en.wikipedia.org/wiki/CLEFIA
https://github.com/pymq/DSA/blob/master/dsa.py
https://pypi.org/project/EDDIE-Tool/
https://en.wikipedia.org/wiki/Elliptic-curve_cryptography
https://en.wikipedia.org/wiki/Claw_finding_problem
https://ec.europa.eu/digital-building-blocks/wikis/display/DIGITAL/Trust+Models+Guidance
https://www.semanticscholar.org/paper/A-Study-of-Encryption-Algorithms-%28RSA%2C-DES%2C-3DES-Singh-Supriya/187d26258dc57d794ce4badb094e64cf8d3f7d88
https://en.wikipedia.org/wiki/Twofish
https://www.thesslstore.com/blog/block-cipher-vs-stream-cipher/
https://www.linkedin.com/learning/learning-cryptography-and-network-security-2/keeping-information-safe?autoplay=true&contextUrn=urn%3Ali%3AlearningCollection%3A6995048663181881344
https://en.wikipedia.org/wiki/ESTREAM
https://infineon.github.io/python-optiga-trust/crypto.html
https://www.linkedin.com/learning/symmetric-cryptography-essential-training/basic-terminology?autoSkip=true&autoplay=true&contextUrn=urn%3Ali%3AlearningCollection%3A6995048663181881344&resume=false
https://www.linkedin.com/learning/comptia-security-plus-sy0-601-cert-prep-3-cryptography-design-and-implementation/cryptography-design-and-implementation?autoplay=true&contextUrn=urn%3Ali%3AlearningCollection%3A6995048663181881344
https://cryptobook.nakov.com/asymmetric-key-ciphers/ecc-encryption-decryption
https://kaliboys.com/wp-content/uploads/2020/11/Implementing-cryptography-using-python.pdf
https://buildmedia.readthedocs.org/media/pdf/pycryptodome/latest/pycryptodome.pdf
Comments