Lets remember some easy calculation, either prime numbers, coprime numbers or triplets are important part of a base for more sophisticated solutions. We can use triplets for arrays and vectorization. Coprime numbers can be used in cryptography, gear wear and circle theories. Prime numbers in Dirichlet prime number theorem or the Riemann hypothesis.
# 1. Triplets
# 1.1 Python code to create triplets from list of words
list_of_words = ['UBS', 'Bank', 'Finance', 'is', 'for']
List = [list_of_words[i:i + 3]
for i in range(len(list_of_words) - 2)]
print(List)
# 1.2 - Python code to find triplet numbers combinations for given sum
from itertools import combinations
def findTriplets(lst, key):
def valid(val):
return sum(val) == key
return list(filter(valid, list(combinations(lst, 3))))
lst = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(findTriplets(lst, 10))
lst = [1, 2, 3, 4, 5]
print(findTriplets(lst, 8))
# 2. Coprime numbers
# 2.1 Coprime numbers True / False
# Two integers are coprime ( = relatively prime, mutually prime ) if their highest common factor HCF ( greatest common divisor GCD ) is only 1
# Coprime pair example - numbers 5 and 9. The factors of 5 are 1 and 5. The factors of 9 are 1, 3, and 9. The factor that is common to both 5 and 9 is 1. GCF of (5, 9) = 1. Thus, (5, 9) is a co-prime pair.
# not Coprime pair - numbers 6 and 10. The factors of 6 are 1, 2, 3, and 6. The factors of 10 are 1, 2, 5, and 10. Factors that are common to both 6 and 10 are 1 and 2. So GCF (6, 10) = 2. Thus, (6, 10) is NOT a co-prime pair.
# Python code to evaluate if two numbers are coprime
def HCF(p,q):
while q != 0:
p, q = q, p%q
return p
def is_coprime(x, y):
return HCF(x, y) == 1
print(is_coprime(5, 9))# True
print(is_coprime(6, 10))# False
print(is_coprime(17, 13))# True
print(is_coprime(17, 21))# True
print(is_coprime(15, 21))# False
print(is_coprime(25, 45))# False
print(is_coprime(13, 93))# True
print(is_coprime(13, 14))# True
# 3. Prime numbers
# 3.1 Prime numbers plotted on polar coordinates
# An integer is prime if fractal without remaining is only 2 numbers: 1 and the number itself
import math
import sympy
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
%config InlineBackend.figure_format='retina'
plt.style.use('seaborn-whitegrid')
# Generate prime numbers
print(list(sympy.primerange(0, 100)))
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
def get_coordinate(num):
return num * np.cos(num), num * np.sin(num)
get_coordinate(1)
(0.5403023058681398, 0.8414709848078965)
x = np.arange(1, 10)
get_coordinate(x)
(array([ 0.54030231, -0.83229367, -2.96997749, -2.61457448, 1.41831093, 5.76102172, 5.27731578, -1.16400027, -8.20017236]),
array([ 0.84147098, 1.81859485, 0.42336002, -3.02720998, -4.79462137, -1.67649299, 4.59890619, 7.91486597, 3.70906637]))
def create_plot(nums, figsize=8, s=None, show_annot=False):
nums = np.array(list(nums))
x, y = get_coordinate(nums)
plt.figure(figsize=(figsize, figsize))
plt.axis("off")
plt.scatter(x, y, s=s, color = 'black')
plt.show()
# If you plot prime numbers to coordinates,it creates spiral. But the pttern of spiral, which is created from positive integeres is still more significant.
# Hence of course, not all the integers are positive.
primes = sympy.primerange(0, 500)
create_plot(primes)
primes = sympy.primerange(0, 20000)
create_plot(primes, s=1)
primes = sympy.primerange(0, 400000)
create_plot(primes, s=0.15)
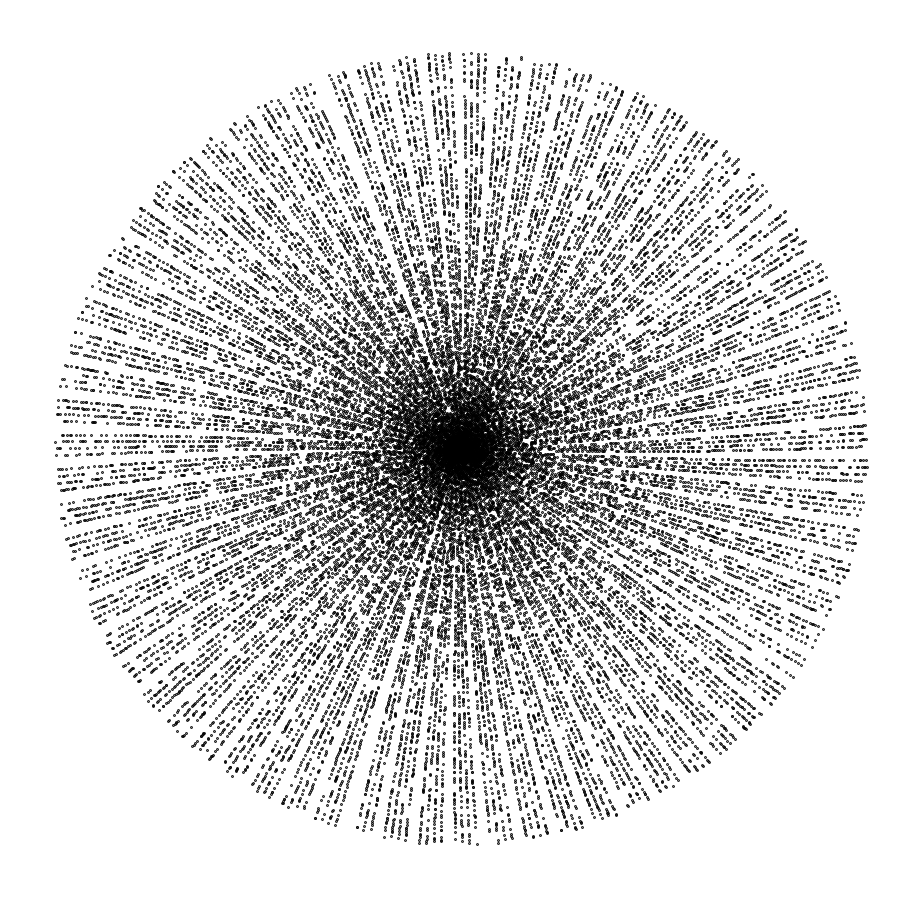
# Range of positive integers in coordintes has more significant spiral
nums = range(1000)
create_plot(nums)
Resources:
Comments